Milan Latinović
Software Engineer and Enterprise Architect
Clean Code Rules: Improve quality of your code

Clean Code Rules is a proposal of common sense rules. They are easy to follow and can improve quality of your code. Therefore, if you work as a developers and architect, you should be aware of them.
Table of Contents
- Introduction
- Clean Code Rules for Design
- Understandability
- Naming
- Clean Code Rules for Functions
- Pro tip: Comments
- Pro tip: Source Code
Introduction
Here is a common use case. You are finished with writing your technical specifications and estimates. Now you need to code this. Yes, you need to make it work.
Final result should be clean code, but it should also fit in your time-frames.
Clean code rules can be summarized is a several simple statements.
Firstly, always follow standard (team defined) conventions. These conventions are created and intended as a standard for each team member. Furthermore, when developer follows a standard other team members can understand his code.
Secondly, there is a “KISS – Keep it Simple Stupid” rule. This rule should be self-explanatory. Don’t over-engineer things. Also don’t over-complicate things. Try to focus on problem at hand and code a solution for it.
Thirdly, always leave the code cleaner than you found it. For example, you can always add missing comments. You can cleanup some complex logic. Maybe write some missing unit tests or simply reduce number of parameters. Make sure that you are not breaking any functionality in a process.
Finally, always try to find a root cause of the problem. By all means, avoid hacking. Avoid making bloated complex code for simple solution. Also avoid making a patches for core instead improving core itself.
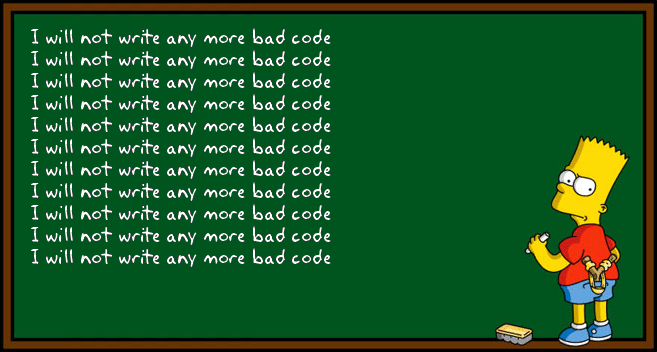
Clean Code Rules for Design
- Keep configurable data at highest levels
- Prefer polymorphism to selections (if/else, switch/case)
- Separate multi-threading code
- Prevent over-configuration
- Use dependency injection
- Understand inheritance (classes and direct dependencies)
Understandability
- Try to code consistently. If you do something in a certain way, do it like that all the time.
- Give meaningful names to variables.
- Try to use objects instead of primitive types, where needed.
- Avoid negative and confusing conditionals.
Naming
- Go for a descriptive and unambiguous names
- Use pronounceable and searchable names
- Replace magic numbers with named constants
- Avoid encodings, such as appending prefixes and type information if not needed
Clean Code Rules for Functions
- Keep functions small
- Follow “Single Responsibility Principle” – Function should do only one thing.
- Functions should have descriptive names.
- Keep the number of arguments in function low.
- Try to avoid side affects of the function.
Pro tip: Comments
- Always try to explain flow or big picture in code.
- Avoid “Anti-pattern commenting” – Try not to comment something that should be obvious from the code itself.
- Follow commenting convention – based on your team rules and programming language (or framework) that you use.
Pro tip: Source Code
- Separate concepts vertically
- Declare variables close to their usages (both horizontally and vertically)
- Keep dependent functions close.
- Keep similar functions close.
- Don’t break indention.
- Keep things simple and pretty.
Do you like my posts? Help me to spread the word. Make one single share with your favorite social network.